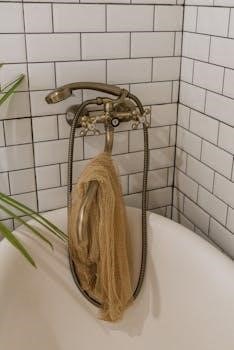
Modern applications, often spread across numerous services, demand robust monitoring. Distributed tracing emerges as a vital technique, offering insights into request flows. It aids in diagnosing performance and functional issues, crucial for maintaining application health. This is especially important in complex microservices-based systems.
What is Distributed Tracing?
Distributed tracing is a methodology for monitoring and understanding the path of a request as it traverses various services within a distributed system; It’s like having a detailed map of how a transaction moves through your application’s architecture. Unlike traditional logging, which focuses on individual components, distributed tracing provides a holistic view, linking together events across different services into a single, coherent trace. This allows developers to follow a user request from its initiation to completion, regardless of how many services it touches. The core idea is to capture data about the request’s journey, including the time spent in each service and any errors encountered. This data is then used to reconstruct the entire request flow, allowing developers to pinpoint bottlenecks and diagnose issues quickly. Distributed tracing is essential for debugging and optimizing complex, microservice-based architectures, providing the visibility needed to ensure reliability and performance.
Why is Distributed Tracing Important?
Distributed tracing is crucial for modern applications due to the complexity and scale of microservices architectures. In such systems, a single user request often involves multiple services, making it difficult to pinpoint performance bottlenecks or failures. Without tracing, identifying the root cause of issues becomes a time-consuming and challenging task. Distributed tracing addresses this problem by providing end-to-end visibility into the request flow. It allows developers to understand how different services interact and where delays occur. This insight is invaluable for optimizing performance and ensuring a smooth user experience. Furthermore, distributed tracing helps in identifying cascading failures, where an issue in one service can propagate to others; By tracking requests across services, developers can quickly isolate and resolve problems before they impact the entire application. It enables faster debugging, improved performance, and increased reliability, making it an indispensable tool for managing complex distributed systems. Overall, it transforms troubleshooting from guesswork to a data-driven process.
.NET and Distributed Tracing
.NET provides built-in support for distributed tracing through the `System.Diagnostics.Activity` API. This allows .NET applications to generate telemetry data for tracking requests across services. Key libraries are instrumented, facilitating automatic trace data collection.
System.Diagnostics.Activity API
The System.Diagnostics.Activity
API serves as the foundation for distributed tracing within .NET applications. Introduced with .NET 5, it incorporates all members of the OpenTelemetry-compliant tracing specification, providing a standardized approach to generating trace data. This API enables developers to create Activity
objects, which represent operations within a distributed trace. These objects store contextual information about the operation, including start and end times, unique IDs, and parent-child relationships, facilitating the reconstruction of request flows. The Activity
API supports both the W3C Trace Context standard, which is the default for .NET 5 and later, and the older Hierarchical ID scheme for backwards compatibility. It allows for the instrumentation of custom code, going beyond the standard libraries, making applications more easily diagnosable. This functionality forms the basis for all distributed tracing at the base class library level in .NET, ensuring consistency in how traces are generated and managed across various components.
W3C Trace Context
The W3C Trace Context standard is crucial for ensuring interoperability in distributed tracing across different systems and services. It provides a standardized format for propagating trace identifiers and context information as requests move through a distributed environment. This standard is the default in .NET 5 and later versions, replacing older, proprietary methods. It defines how trace IDs and span IDs are generated and how they are passed along in HTTP headers, enabling the reconstruction of entire request paths. By using W3C Trace Context, disparate services, regardless of the technology they use, can correlate their activities within a single distributed trace. This ensures that a request that traverses multiple applications can be tracked seamlessly. The adoption of this standard promotes a more universal approach to distributed tracing, making it easier to analyze complex systems. It also simplifies integrating various tools and services into a coherent tracing solution.
.NET Libraries Instrumentation
Many key .NET libraries are already instrumented to automatically generate distributed tracing data. This built-in instrumentation reduces the need for manual code additions and provides a solid foundation for monitoring application behavior. Libraries such as those used for HTTP communication, database access, and message queuing, include code that creates Activity objects, which represent spans in a distributed trace. However, while core libraries are instrumented, developers might need to add custom instrumentation to capture application-specific details. This custom instrumentation enhances diagnosability by providing greater insight into internal application logic. Using the System.Diagnostics.Activity
API, developers can create their own spans to track specific operations or methods. This allows for a detailed view of the application’s internal workings, making it easier to pinpoint performance bottlenecks or errors. Combining the built-in instrumentation with custom code provides a comprehensive view of the application’s activity within distributed systems.
OpenTelemetry and .NET
OpenTelemetry (OTel) is a vendor-neutral, open-source framework crucial for observability. It provides APIs, SDKs, and tools to generate telemetry data, including traces. OTel enables a unified way to collect and manage this data in .NET applications.
OpenTelemetry for .NET
OpenTelemetry provides a standardized approach to instrumenting .NET applications for distributed tracing. It offers libraries and SDKs that allow developers to add instrumentation to their code, automatically capturing trace, metrics, and log data. This framework is vendor-neutral, ensuring that telemetry data can be exported to various backends like Jaeger. The integration simplifies the process of collecting valuable insights from complex systems. With OpenTelemetry, .NET developers can achieve comprehensive observability without being locked into a specific vendor’s solution. The unified approach helps in better understanding the behavior and health of distributed applications. Furthermore, it streamlines the collection of telemetry data across different services, enabling a holistic view of the system’s performance. By using the provided instrumentation, developers can easily pinpoint bottlenecks and performance issues in their .NET applications. This ultimately leads to faster debugging and improved application reliability, making OpenTelemetry an essential tool for modern .NET development.
OpenTelemetry Configuration in .NET
Configuring OpenTelemetry in .NET involves adding specific NuGet packages and setting up services to collect and export telemetry data. Developers typically install packages for tracing, metrics, and logging, along with an exporter to send data to a chosen backend. For example, to export traces to Jaeger, the `OpenTelemetry.Exporter.Jaeger` package is used. The configuration includes specifying the endpoint where the telemetry data should be sent, often through environment variables or application settings. The OTEL_EXPORTER_OTLP_ENDPOINT environment variable is commonly used for configuring the OpenTelemetry Protocol (OTLP) exporter, pointing to the Jaeger instance. Instrumentations are also configured, enabling automatic capture of traces from various libraries and frameworks. This setup allows applications to generate and export trace data, providing essential visibility into distributed systems. Proper configuration ensures that all relevant telemetry is collected, facilitating performance analysis and issue resolution. This streamlined approach makes it easier to manage and understand the behavior of .NET applications.
Implementing Distributed Tracing
Implementing distributed tracing involves collecting trace data, storing it centrally, and then analyzing it. This requires instrumenting applications, configuring exporters, and selecting a suitable backend. Proper configuration is essential for effective monitoring and troubleshooting of distributed systems.
Collecting and Storing Trace Data
The process of collecting and storing trace data is fundamental to distributed tracing’s effectiveness. Initially, .NET applications are instrumented using the System.Diagnostics.Activity
API to generate telemetry data. This API creates Activity
objects that represent operations within a distributed trace. However, this data must be gathered and stored in a centralized location for later analysis. Key .NET libraries are already instrumented to automatically produce this information. Developers often choose a telemetry service to store the trace data and use a corresponding library to transmit it. For storage, distributed storage systems like Cassandra, Elasticsearch, or HBase are often used, which provide scalability and fault tolerance. Cloud-based solutions, such as AWS X-Ray or Google Cloud Trace, can also be used for this purpose. It’s crucial to ensure that the tracing backend can handle the data volume generated by the application, potentially necessitating powerful hardware or cloud solutions. Configuration of the exporter is also crucial for data to be sent correctly.
Analyzing Distributed Traces
Analyzing distributed traces is essential for understanding the behavior of complex systems. Once trace data is collected and stored, it can be visualized and analyzed using various tools. These tools typically present traces as flame graphs, which show the sequence of service calls and the time spent in each service. This allows developers to pinpoint performance bottlenecks and identify the root cause of issues. By examining the trace data, one can track requests across multiple services, observing how they interact and where delays may occur. Detailed information, such as SQL queries executed or specific HTTP requests, is also available, providing valuable context. Analyzing traces can also help to understand the flow of data through a distributed system. Furthermore, distributed traces can include contextual information useful for debugging. Effective analysis of distributed traces enables developers to optimize system performance, resolve production issues faster, and gain a deeper understanding of their application’s architecture. OpenTelemetry plays a key role in making this analysis possible.
Jaeger as a Tracing Backend
Jaeger is an open-source, distributed tracing platform that serves as a powerful backend for storing and visualizing trace data. It plays a crucial role in understanding how requests flow through complex, distributed systems. Jaeger allows developers to map the path of requests as they traverse multiple services. By collecting trace spans from applications instrumented with OpenTelemetry, Jaeger pieces together a complete picture of a transaction. The platform’s user interface provides a visual representation of these traces, allowing for analysis of performance and identification of bottlenecks. Jaeger excels at correlating data from various sources and provides insights into the interaction between different services. Furthermore, it offers features for searching and filtering traces, allowing developers to focus on specific areas of interest. The platform is designed to be scalable and handle large volumes of trace data. It integrates well with other tools and technologies, making it a valuable asset in any modern development environment. Setting up Jaeger is relatively simple, often using Docker containers.